Rotate image by specified angle and output will create a new graphics area on which input image will be placed as rotated but its size, quality and scaling is not changed.
This is helpful when you want to output image in enlarged size while containing input image with its original size.
This will also take care about different DPI sizes while rotation, as images generated from different operating systems have different DPI values. Following will also sync. this with existing DPI capabilities...
You may also provide background color for output image.
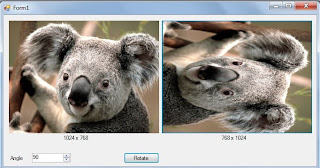
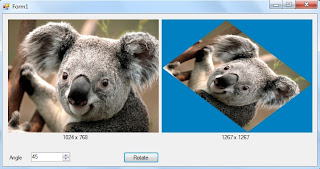
public byte[] RotateImage(string imgFile, float rotationAngle, Color BGColor)
{
Image img = Image.FromFile(imgFile);
Bitmap bmpTemp;
Graphics gfx4DPI = Graphics.FromImage(new Bitmap(100, 100));
bmpTemp = new Bitmap(Convert.ToInt32(img.Width * gfx4DPI.DpiX / img.HorizontalResolution), Convert.ToInt32(img.Height * gfx4DPI.DpiY / img.VerticalResolution));
gfx4DPI.Dispose();
Graphics gfxTemp = Graphics.FromImage(bmpTemp);
//now we set the rotation point to the center of our image
gfxTemp.TranslateTransform((float)(bmpTemp.Width) / 2, (float)(bmpTemp.Height) / 2);
gfxTemp.RotateTransform(rotationAngle);
//start actual here
Bitmap bmp = new Bitmap(Convert.ToInt32(gfxTemp.VisibleClipBounds.Width), Convert.ToInt32(gfxTemp.VisibleClipBounds.Height));
gfxTemp.Dispose();
//bmpTemp.Dispose();
Graphics gfx = Graphics.FromImage(bmp);
Rectangle bgImg = new Rectangle(0, 0, bmp.Width, bmp.Height);
SolidBrush bgBrush = new SolidBrush(BGColor);
gfx.FillRegion(bgBrush, new Region(bgImg));
gfx.TranslateTransform((float)(bmp.Width) / 2, (float)(bmp.Height) / 2);
gfx.RotateTransform(rotationAngle);
gfx.TranslateTransform(-1 * (float)(bmpTemp.Width) / 2, -1 * (float)(bmpTemp.Height) / 2);
gfx.ScaleTransform((float)0.99, (float)0.99);
//set the InterpolationMode to HighQualityBicubic so to ensure a high
//quality image once it is transformed to the specified size
gfx.InterpolationMode = InterpolationMode.HighQualityBicubic;
//now draw our new image onto the graphics object
gfx.DrawImage(img, new Point(0, 0));
gfx.Dispose();
return imageToByteArray(bmp);
}
private byte[] imageToByteArray(System.Drawing.Image imageIn)
{
MemoryStream ms = new MemoryStream();
imageIn.Save(ms, System.Drawing.Imaging.ImageFormat.Gif);
return ms.ToArray();
}
This comment has been removed by the author.
ReplyDelete